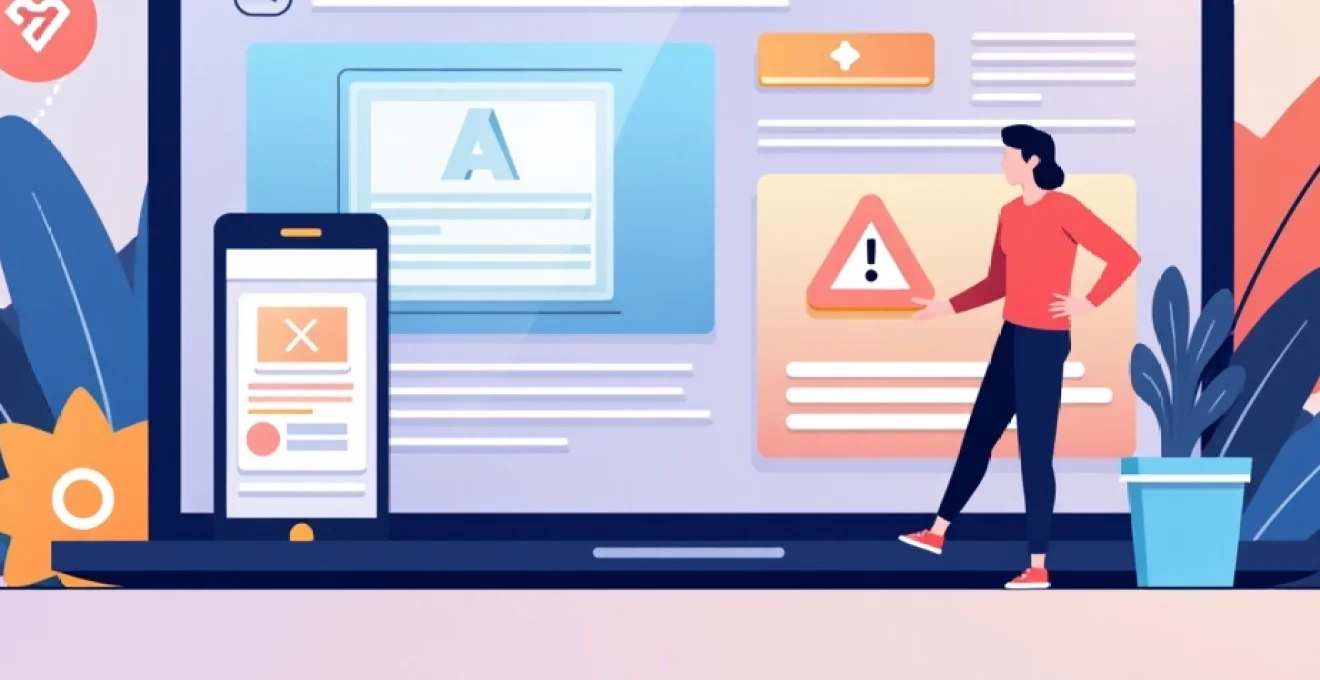
In today's digital landscape, the proliferation of mobile devices has fundamentally changed how users interact with websites. As smartphones and tablets become the primary means of accessing the internet for many, the importance of responsive development in creating mobile-friendly websites cannot be overstated. Responsive web design ensures that your site looks and functions seamlessly across a wide range of devices, from large desktop monitors to small smartphone screens.
By embracing responsive development, you're not just catering to user preferences; you're also aligning with search engine best practices, improving your site's performance, and potentially boosting your online visibility. Let's dive deep into the world of responsive web design and explore why it's crucial for modern web development.
Fundamentals of responsive web design
Responsive web design is an approach that makes web pages render well on a variety of devices and window or screen sizes. It's about creating websites that provide an optimal viewing experience—easy reading and navigation with a minimum of resizing, panning, and scrolling—across a wide range of devices.
At its core, responsive design relies on three main principles:
- Fluid grids
- Flexible images
- Media queries
These principles work together to create a flexible and adaptable layout that responds to the user's viewport. By using relative units like percentages instead of fixed pixels, elements can resize proportionally. Flexible images ensure that visual content doesn't break the layout, while media queries allow for different styles to be applied based on the device's characteristics.
Implementing these fundamentals requires a shift in thinking from fixed, pixel-perfect designs to more fluid and adaptable layouts. It's about creating a single website that works everywhere, rather than multiple versions for different devices.
Mobile-first approach and its impact on development
The mobile-first approach is a design strategy that prioritizes designing for mobile devices before scaling up to larger screens. This methodology has gained significant traction in recent years, largely due to the increasing dominance of mobile traffic. By starting with the mobile experience, developers are forced to focus on the essential elements of their website, leading to cleaner, more efficient designs.
Adopting a mobile-first approach can have a profound impact on the development process. It encourages developers to think critically about content prioritization, performance optimization, and user experience from the outset. This approach often results in faster, more streamlined websites that perform well across all devices.
Progressive enhancement vs. graceful degradation
When it comes to implementing responsive design, two contrasting philosophies emerge: progressive enhancement and graceful degradation. Progressive enhancement starts with a basic, functional website and then adds more advanced features for devices that can support them. Conversely, graceful degradation begins with a fully-featured website and then scales back functionality for less capable devices.
Progressive enhancement aligns well with the mobile-first approach, as it ensures that the core content and functionality are accessible to all users, regardless of their device capabilities. This method often leads to more robust, inclusive designs that work well across a broader range of devices and browsers.
Implementing mobile-first with CSS media queries
CSS media queries are a powerful tool for implementing responsive design, particularly when taking a mobile-first approach. They allow you to apply different styles based on the characteristics of the device, such as screen width, height, or resolution.
Here's a basic example of how you might use media queries in a mobile-first approach:
/* Base styles for mobile */body { font-size: 16px;}/* Styles for larger screens */@media screen and (min-width: 768px) { body { font-size: 18px; }}
In this example, we start with base styles suitable for mobile devices and then use a media query to apply different styles when the screen width is at least 768 pixels. This approach ensures that mobile devices receive the simplest, most performant styles by default.
Performance optimization for mobile devices
Performance is crucial for mobile-friendly websites. Mobile users often have slower internet connections and less powerful devices, making optimization essential. Some key strategies for mobile performance optimization include:
- Minimizing HTTP requests
- Optimizing images for mobile
- Using efficient CSS and JavaScript
- Implementing lazy loading for images and content
- Leveraging browser caching
By focusing on performance from the start, you can ensure that your responsive website provides a smooth, enjoyable experience for all users, regardless of their device or connection speed.
Fluid grids and flexible layouts
Fluid grids are a cornerstone of responsive web design. Unlike fixed-width layouts, fluid grids use relative units (like percentages) instead of absolute units (like pixels). This allows the layout to adapt smoothly to different screen sizes.
Creating a fluid grid involves dividing the layout into columns and setting their widths as percentages of the container. This approach ensures that the layout maintains its proportions as the screen size changes, providing a consistent user experience across devices.
CSS Grid vs. Flexbox for responsive layouts
Both CSS Grid and Flexbox are powerful tools for creating responsive layouts, each with its own strengths. CSS Grid is ideal for two-dimensional layouts, allowing you to create complex grid structures with ease. Flexbox, on the other hand, excels at one-dimensional layouts and is particularly useful for aligning and distributing space among items in a container.
Here's a simple comparison of CSS Grid and Flexbox:
Feature | CSS Grid | Flexbox |
---|---|---|
Dimensional Control | Two-dimensional | One-dimensional |
Layout Type | Grid-based | Linear-based |
Alignment | Both row and column | Main axis and cross axis |
In practice, many responsive designs use a combination of both CSS Grid and Flexbox, leveraging the strengths of each to create flexible, robust layouts.
Relative units (em, rem, vw, vh) in responsive design
Relative units play a crucial role in creating truly responsive designs. Unlike absolute units like pixels, relative units scale with the size of the viewport or the root element, allowing for more flexible and adaptable layouts. Some key relative units include:
em
: Relative to the font size of the parent elementrem
: Relative to the font size of the root elementvw
: 1% of the viewport widthvh
: 1% of the viewport height
Using these units effectively can help create layouts that scale smoothly across different screen sizes, enhancing the overall responsiveness of your website.
Creating responsive images with srcset and sizes attributes
Images often pose a challenge in responsive design, as they need to look good on both small mobile screens and large desktop displays. The srcset
and sizes
attributes provide a powerful solution to this problem, allowing you to specify multiple image sources for different screen sizes and resolutions.
This code tells the browser to use different image sources based on the viewport width, ensuring that users always receive an appropriately sized image for their device.
Responsive framework integration
While it's possible to build responsive websites from scratch, many developers choose to leverage responsive frameworks to speed up development and ensure consistency. These frameworks provide pre-built components and grid systems that are designed to be responsive out of the box.
Bootstrap 5's responsive features and grid system
Bootstrap is one of the most popular responsive frameworks, known for its comprehensive set of components and powerful grid system. Bootstrap 5, the latest major version, offers a mobile-first, responsive grid system that uses a series of containers, rows, and columns to layout and align content.
Bootstrap's grid system is based on a 12-column layout and includes five default responsive tiers. This allows you to create complex, responsive layouts with minimal custom CSS. Additionally, Bootstrap provides a wide range of responsive utility classes for quickly toggling common values such as display, margin, and padding.
Tailwind CSS utility classes for responsive design
Tailwind CSS takes a different approach to responsive design, offering a utility-first CSS framework. Instead of pre-styled components, Tailwind provides low-level utility classes that you can combine to build custom designs.
Tailwind's responsive design system is based on customizable breakpoints and modifier classes. For example, you might use classes like md:flex lg:w-1/2
to create a layout that's full-width on mobile, half-width on larger screens, and uses flexbox on medium-sized screens and up.
Foundation framework's responsive components
Foundation is another popular responsive framework, known for its flexibility and advanced features. It offers a responsive grid system, as well as a wide range of responsive components like navigation bars, forms, and modals.
One of Foundation's strengths is its emphasis on semantic markup. It allows you to create responsive layouts using semantic HTML classes, which can lead to more maintainable and accessible code.
Advanced responsive techniques
As responsive web design has evolved, more advanced techniques have emerged to tackle complex design challenges and improve user experiences across devices.
Implementing responsive typography with CSS clamp()
Responsive typography is crucial for maintaining readability across different screen sizes. The clamp()
function in CSS provides a powerful tool for creating fluid typography that scales smoothly with the viewport size.
Here's an example of how you might use clamp()
for responsive font sizes:
body { font-size: clamp(1rem, 2.5vw, 2rem);}
This sets the font size to be at least 1rem, at most 2rem, and 2.5% of the viewport width in between these limits. This creates a smooth, responsive scaling effect that ensures text remains readable at all screen sizes.
Container queries: the future of component-level responsiveness
While media queries have been the backbone of responsive design for years, they have limitations when it comes to reusable components. Container queries aim to solve this by allowing styles to be applied based on the size of a containing element, rather than the viewport.
Although container queries are not yet widely supported, they represent an exciting future direction for responsive design. They will allow for truly modular, context-aware components that can adapt their layout based on their container, regardless of the overall page layout.
Responsive design patterns: Off-Canvas, Column Drop, Layout Shifter
Several common responsive design patterns have emerged to solve specific layout challenges:
- Off-Canvas: This pattern moves less frequently used content (like navigation or app menus) off-screen, showing it only when requested. It's particularly useful for complex navigation on small screens.
- Column Drop: As the screen width gets narrower, this pattern simply stacks columns vertically. It's a straightforward way to handle multi-column layouts on smaller screens.
- Layout Shifter: This advanced pattern uses different layouts for different screen sizes, often changing both the size and position of elements. It provides the most control but can be more complex to implement.
These patterns can be mixed and matched to create responsive designs that work well across a wide range of devices and screen sizes.
Testing and optimization for mobile-friendly websites
Creating a responsive design is only part of the process; thorough testing and optimization are crucial to ensure your website performs well on all devices.
Chrome DevTools Device Mode for responsive testing
Chrome DevTools offers a powerful Device Mode that allows you to simulate various mobile devices and test your responsive designs. You can toggle device emulation, adjust screen size and orientation, and even simulate different network conditions.
Using Device Mode, you can quickly identify and fix issues with your responsive layout, ensuring that your design works well across a wide range of devices.
Lighthouse performance audits for mobile optimization
Lighthouse, an open-source tool from Google, provides automated audits for performance, accessibility, progressive web apps, and more. It's particularly useful for optimizing mobile performance.
Running a Lighthouse audit on your mobile site can provide valuable insights into areas for improvement, such as image optimization, render-blocking resources, and first contentful paint times. These insights can help you fine-tune your site for optimal mobile performance.
Cross-browser testing with BrowserStack and Sauce Labs
While Chrome DevTools is excellent for initial testing, it's crucial to test your responsive design across multiple browsers and real devices. Services like BrowserStack and Sauce Labs provide cloud-based testing environments that allow you to test your site on a wide range of real devices and browsers.
These tools can help you identify and fix browser-specific issues, ensuring that your responsive design works well for all users, regardless of their preferred browser or device.
By thoroughly testing and optimizing your responsive design, you can ensure that your website provides an excellent user experience across all devices, from the smallest smartphones to the largest desktop monitors. This comprehensive approach to responsive development is key to creating truly mobile-friendly websites that meet the needs of today's diverse web users.